PuppeteerでAmazonアフィリエイトのレポートを取得する
更新日:2022.07.02
作成日:2017.08.22
GoogleChrome/puppeteer: Headless Chrome Node API というHeadless Chromeを操作するJavaScriptライブラリが出たので使ってみました。
何度も実行してロボット判定されてしまったので、実際にはHeadlessで動作確認していないが(おそらく)こんな感じで取得できるはず。
2017/09/10追記
デバッグモード以外では毎回ロボット判定されてしまうため、Cookieからログインする方法に変えました。 初回ログイン時は、デバッグモードで動かし、Cookieを保存し、2回目以降はCookieからログインする。
インストール
yarn add puppeteer
コード
const puppeteer = require('puppeteer');
const fs = require('fs');
const cookies_path = './cookies_amazon.json';
const {AMAZON_ID, AMAZON_PW} = require('./config');
/**
* debugモードでログイン
*/
async function login(page) {
await page.goto('https://affiliate.amazon.co.jp/');
await page.click('#a-autoid-0-announce');
await page.waitFor(2000);
await page.focus('#ap_email');
await page.type(AMAZON_ID);
await page.waitFor(2000);
await page.focus('#ap_password');
await page.type(AMAZON_PW);
await page.waitFor(2000);
await page.click('#signInSubmit');
await page.waitFor(5000);
await page.screenshot({path: 'images/amazon_logined.png'});
}
/**
* Cookieを利用してログイン
*/
async function loginWithCookie(page) {
const cookies = JSON.parse(fs.readFileSync(cookies_path, 'utf-8'));
for (let cookie of cookies) {
await page.setCookie(cookie);
}
}
(async () => {
const browser = await puppeteer.launch({
headless: true,
});
const page = await browser.newPage();
await page.setViewport({width: 1280, height: 700});
// await login(page);
await loginWithCookie(page);
await page.goto('https://affiliate.amazon.co.jp/home');
await page.screenshot({path: 'images/amazon_login.png'});
await page.goto('https://affiliate.amazon.co.jp/home/reports?ac-ms-src=summaryforthismonth');
await page.waitForSelector('#ac-report-commission-commision-total');
const commission = await page.evaluate(() => {
return document.querySelector('#ac-report-commission-commision-total').textContent;
});
console.log(commission);
await page.screenshot({path: 'images/amazon_after.png'});
const afterCookies = await page.cookies();
fs.writeFileSync('cookies_amazon.json', JSON.stringify(afterCookies));
browser.close();
})();
Cookie操作
Cookie保存
const cookies = await page.cookies();
fs.writeFileSync('cookies_amazon.json', JSON.stringify(cookies));
Cookie取得・設定
const cookies_path = './cookies_amazon.json';
const cookies = JSON.parse(fs.readFileSync(cookies_path, 'utf-8'));
for (let cookie of cookies) {
await page.setCookie(cookie);
}
こんな感じで保存したCookieを設定してあげることで、2回目以降Cookieを使ってログインできました。
参考
Related contents
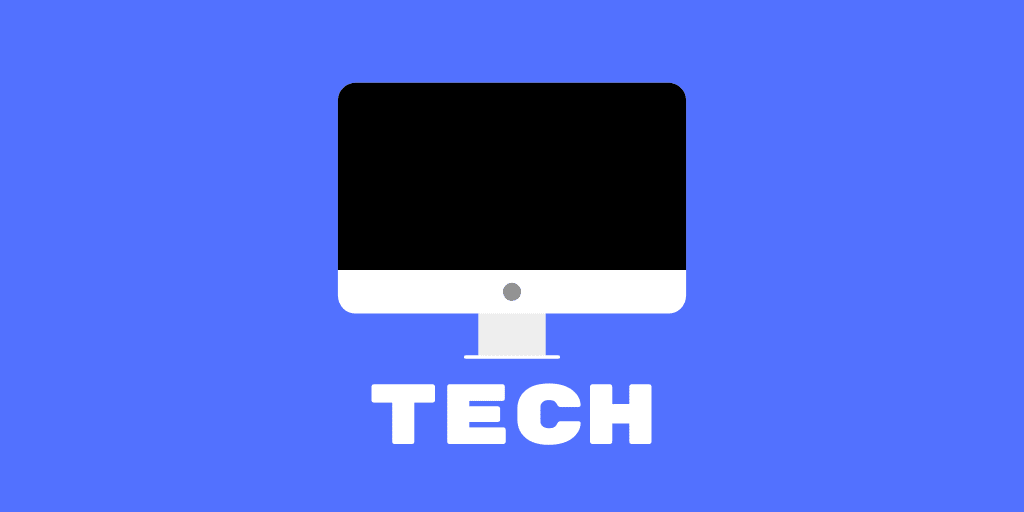
TECH
2020.07.04
puppeteerをAWS Lambdaで利用する
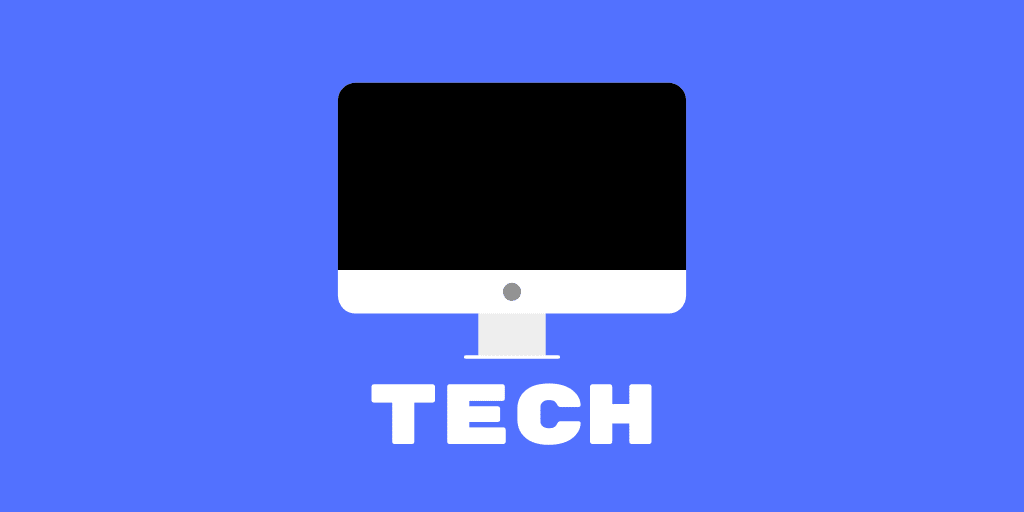
TECH
2020.04.20
Puppeteerを使ってQrunchへクロス投稿する
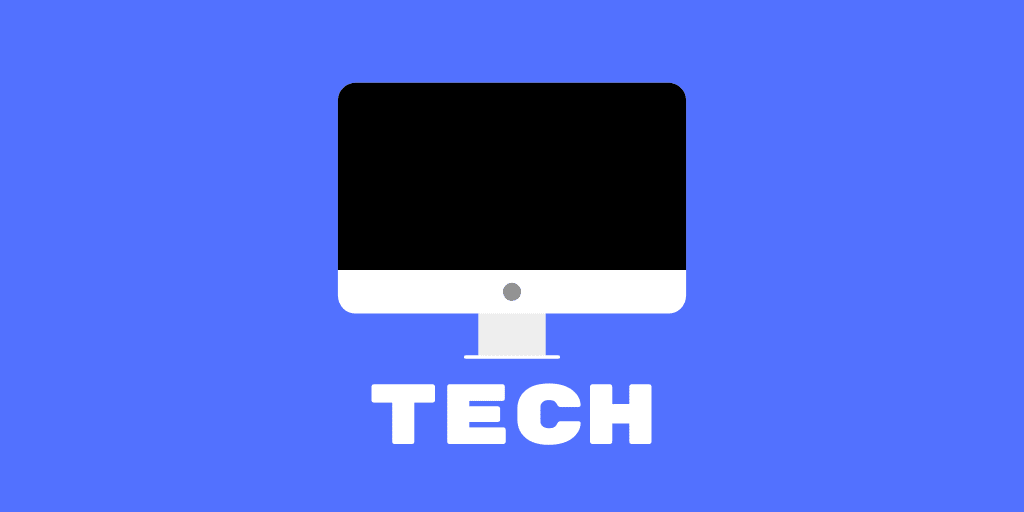
TECH
2020.01.30
PuppeteerのwaitForNavigationで正しくページ遷移を待つ